Axios 详解
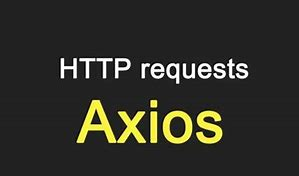
1. 简介
- 定义:Axios 是一个基于 Promise 的 HTTP 客户端,用于浏览器和 Node.js 环境,简化 HTTP 请求的发送和处理。
- 核心特点:
- 支持 Promise API,可链式调用。
- 自动转换 JSON 数据。
- 支持请求/响应拦截。
- 可取消请求。
- 客户端支持防抖和跨域请求。
2. 核心功能
功能 | 描述 |
---|
GET/POST 请求 | 发送 GET 、POST 等 HTTP 方法请求。 |
Promise 支持 | 通过 .then() 和 .catch() 处理异步操作。 |
拦截器 | 在请求发送前或响应返回后拦截并修改请求/响应数据。 |
取消请求 | 通过 CancelToken 取消未完成的请求。 |
响应类型 | 支持 response.data 、response.status 等详细响应信息。 |
3. 基本用法示例
npm install axios
axios.get('/api/users', {params: { id: 1 }
})
.then(response => {console.log(response.data);
})
.catch(error => {console.error('Error:', error);
});
axios.post('/api/create', {name: 'Alice',age: 25
}, {headers: { 'Content-Type': 'application/json' }
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
4. 配置选项
配置项 | 描述 |
---|
baseURL | 请求的基础路径(全局或实例配置)。 |
timeout | 请求超时时间(毫秒)。 |
headers | 自定义请求头(如 Authorization )。 |
withCredentials | 是否允许跨域请求携带凭证(如 Cookie)。 |
paramsSerializer | 自定义参数序列化函数(如处理对象为 URL 参数)。 |
axios.defaults.baseURL = 'https://api.example.com';
axios.defaults.timeout = 5000;
5. 拦截器
-
请求拦截器:在请求发送前修改配置:
axios.interceptors.request.use(config => {config.headers.Authorization = `Bearer ${localStorage.token}`;return config;
}, error => Promise.reject(error));
-
响应拦截器:在响应返回后处理数据或错误:
axios.interceptors.response.use(response => {return response.data;
}, error => {if (error.response.status === 401) {}return Promise.reject(error);
});
6. 错误处理
- 常见错误类型:
error.response
:包含状态码和响应数据(如 404、500)。error.request
:请求已发送但无响应。error.message
:其他错误信息(如网络问题)。
axios.get('/api/invalid')
.catch(error => {if (error.response) {console.log('Status:', error.response.status);console.log('Error Data:', error.response.data);} else {console.log('Request Failed:', error.message);}
});
7. 与 Fetch 的对比
特性 | Axios | Fetch |
---|
Promise 支持 | 原生支持 | 原生支持 |
请求/响应拦截 | 支持 | 不支持 |
取消请求 | 支持 | 需通过 AbortController 实现 |
JSON 自动解析 | 自动转换 | 需调用 response.json() |
浏览器兼容性 | 兼容性好(需 polyfill 旧版浏览器) | 需 polyfill IE 等旧版浏览器 |
8. 常见场景
- 跨域请求:通过
CORS
配置或代理解决(如在开发环境配置代理)。 - 上传文件:
const file = document.querySelector('input[type="file"]').files[0];
const formData = new FormData();
formData.append('file', file);
axios.post('/api/upload', formData, {headers: { 'Content-Type': 'multipart/form-data' }
});
9. 最佳实践
- 避免全局配置污染:通过
axios.create()
创建实例,隔离不同环境配置。 - 统一错误处理:通过拦截器集中处理 Token 过期、网络错误等通用问题。
- 取消重复请求:在组件卸载时取消未完成的请求,避免内存泄漏。
- 合理设置超时:根据接口复杂度调整
timeout
防止阻塞。
const apiClient = axios.create({baseURL: '/api',timeout: 10000
});