安装 Newtonsoft.Json
Install-Package Newtonsoft.Json
代码
HttpClient client = new HttpClient();
HttpResponseMessage response = client.GetAsync("https://opentdb.com/api.php?amount=10&category=18&difficulty=easy&type=multiple").Result;
response.EnsureSuccessStatusCode();
string content = response.Content.ReadAsStringAsync().Result;
Dictionary<string, object> dic = JsonConvert.DeserializeObject<Dictionary<string, object>>(content);
List<Result> results = JsonConvert.DeserializeObject<List<Result>>(dic["results"].ToString());
foreach(Result result in results)
{
result.incorrect_answers.ForEach(item =>Console.WriteLine(item));
}
Result类
internal class Result
{
public string type;
public string difficulty;
public string category;
public string question;
public string correct_answer;
public List<string> incorrect_answers;
}
winform代码
using Newtonsoft.Json;
namespace WinFormsApp1
{
public partial class Form1 : Form
{
List<Result> results = new List<Result>();
int index = 0;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
HttpClient client = new HttpClient();
HttpResponseMessage response = client.GetAsync("https://opentdb.com/api.php?amount=10&category=18&difficulty=easy&type=multiple").Result;
response.EnsureSuccessStatusCode();
string content = response.Content.ReadAsStringAsync().Result;
Dictionary<string, object> dic = JsonConvert.DeserializeObject<Dictionary<string, object>>(content);
results = JsonConvert.DeserializeObject<List<Result>>(dic["results"].ToString());
this.label1.Text = results[index].question;
List<string> tmpList = new List<string>();
tmpList.Add(results[index].correct_answer);
tmpList.Add(results[index].incorrect_answers[0]);
tmpList.Add(results[index].incorrect_answers[1]);
tmpList.Add(results[index].incorrect_answers[2]);
Random random = new Random();
tmpList.Sort((a, b) => random.Next(-1, 2));
this.radioButton1.Text = tmpList[0];
this.radioButton2.Text = tmpList[1];
this.radioButton3.Text = tmpList[2];
this.radioButton4.Text = tmpList[3];
index++;
this.button1.Enabled = (index < results.Count);
this.radioButton1.ForeColor = Color.Black;
this.radioButton2.ForeColor = Color.Black;
this.radioButton3.ForeColor = Color.Black;
this.radioButton4.ForeColor = Color.Black;
this.radioButton1.Checked = false;
this.radioButton2.Checked = false;
this.radioButton3.Checked = false;
this.radioButton4.Checked = false;
}
private void button1_Click(object sender, EventArgs e)
{
this.label1.Text = results[index].question;
List<string> tmpList = new List<string>();
tmpList.Add(results[index].correct_answer);
tmpList.Add(results[index].incorrect_answers[0]);
tmpList.Add(results[index].incorrect_answers[1]);
tmpList.Add(results[index].incorrect_answers[2]);
Random random = new Random();
tmpList.Sort((a, b) => random.Next(-1, 2));
this.radioButton1.Text = tmpList[0];
this.radioButton2.Text = tmpList[1];
this.radioButton3.Text = tmpList[2];
this.radioButton4.Text = tmpList[3];
index++;
this.button1.Enabled = (index < results.Count);
this.radioButton1.ForeColor = Color.Black;
this.radioButton2.ForeColor = Color.Black;
this.radioButton3.ForeColor = Color.Black;
this.radioButton4.ForeColor = Color.Black;
this.radioButton1.Checked = false;
this.radioButton2.Checked = false;
this.radioButton3.Checked = false;
this.radioButton4.Checked = false;
}
private void showCorrectAnswer()
{
if (this.radioButton1.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton1.ForeColor = Color.Blue;
}
if (this.radioButton2.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton2.ForeColor = Color.Blue;
}
if (this.radioButton3.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton3.ForeColor = Color.Blue;
}
if (this.radioButton4.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton4.ForeColor = Color.Blue;
}
}
private void radioButton1_Click(object sender, EventArgs e)
{
if (this.radioButton1.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton1.ForeColor = Color.Blue;
}
else
{
this.radioButton1.ForeColor = Color.Red;
showCorrectAnswer();
}
}
private void radioButton2_Click(object sender, EventArgs e)
{
if (this.radioButton2.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton2.ForeColor = Color.Blue;
}
else
{
this.radioButton2.ForeColor = Color.Red;
showCorrectAnswer();
}
}
private void radioButton3_Click(object sender, EventArgs e)
{
if (this.radioButton3.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton3.ForeColor = Color.Blue;
}
else
{
this.radioButton3.ForeColor = Color.Red;
showCorrectAnswer();
}
}
private void radioButton4_Click(object sender, EventArgs e)
{
if (this.radioButton4.Text.Equals(this.results[index - 1].correct_answer))
{
this.radioButton4.ForeColor = Color.Blue;
}
else
{
this.radioButton4.ForeColor = Color.Red;
showCorrectAnswer();
}
}
}
}
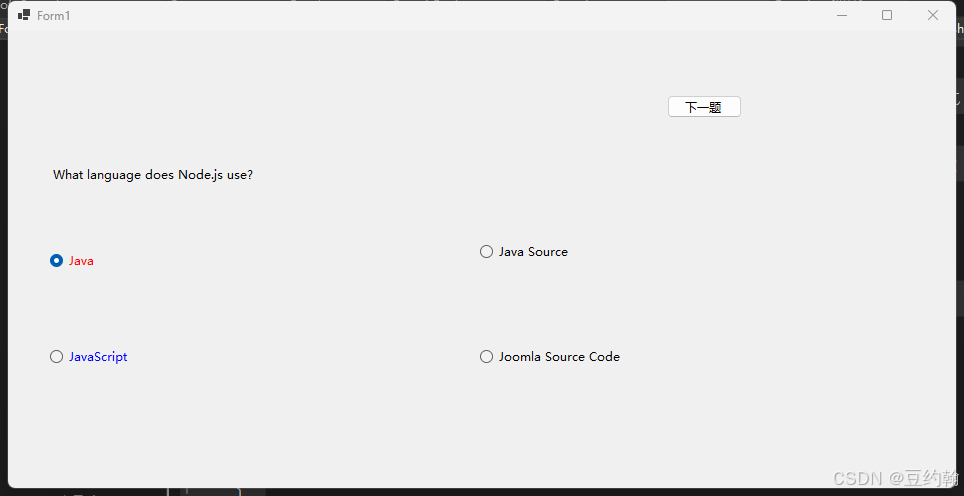