spring框架学习(下)
这章节讲的主要是spring在生产Bean对象时的过程
Spring实例化对象的基本流程
1、解析bean.xml
2、封装成BeanDifinition类
3、存放到BeanDIfinitionMap里
4、从BeanDIfinitionMap遍历得到bean
5、将bean存放到SingletonObjects
6、调用getBean方法得到bean
以下是简易的流程图:
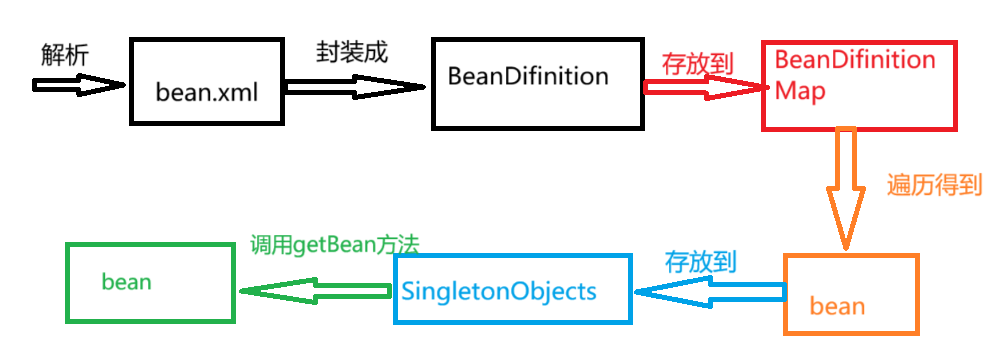
1、Bean的处理器(xxxxPostProcessor)
基础概念:Spring的后处理是Spring对外开发的重要扩展点,它允许我们介入到Bean的整个实例化流程中来,可以动态添加、修改BeanDefinition、动态修改Bean。
Spring主要有两种后处理器:一种是Bean工厂后处理器(BeanFactoryPostProcessor)另一种是Bean后处理器(BeanPostProcessor)。
区别在于:
Bean工厂后处理器在BeanDefinitionMap填充完毕,Bean实例化之前执行;
Bean后处理器一般在bean实例化后,填充到单例池SingletonObjectes之前执行;
但是我还没有研究透彻,还不能很好的说明,所以就先跳过这个知识点
2、Bean的生命周期
Spring Bean 的生命周期可以概括为以下几个主要阶段:
- 实例化:容器借助反射机制创建 Bean 的实例。
- 属性赋值:为 Bean 的属性注入值,这些值可能来自配置文件、注解等。
- 初始化:执行初始化方法,例如实现
InitializingBean
接口的afterPropertiesSet
方法,或者使用@PostConstruct
注解的方法。 - 使用:Bean 被应用程序使用。
- 销毁:执行销毁方法,例如实现
DisposableBean
接口的destroy
方法,或者使用@PreDestroy
注解的方法。
import org.springframework.beans.factory.DisposableBean;
import org.springframework.beans.factory.InitializingBean;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;// 定义一个 Bean 类
public class MyBean implements InitializingBean, DisposableBean {private String message;// 构造函数,用于实例化 Beanpublic MyBean() {System.out.println("MyBean: 实例化");}// 属性赋值方法public void setMessage(String message) {this.message = message;System.out.println("MyBean: 属性赋值 - message = " + message);}// 使用 @PostConstruct 注解的初始化方法@PostConstructpublic void postConstruct() {System.out.println("MyBean: @PostConstruct 注解的初始化方法");}// 实现 InitializingBean 接口的 afterPropertiesSet 方法@Overridepublic void afterPropertiesSet() throws Exception {System.out.println("MyBean: InitializingBean 接口的 afterPropertiesSet 方法");}// 自定义的初始化方法public void initMethod() {System.out.println("MyBean: 自定义的初始化方法");}// 使用 @PreDestroy 注解的销毁方法@PreDestroypublic void preDestroy() {System.out.println("MyBean: @PreDestroy 注解的销毁方法");}// 实现 DisposableBean 接口的 destroy 方法@Overridepublic void destroy() throws Exception {System.out.println("MyBean: DisposableBean 接口的 destroy 方法");}// 自定义的销毁方法public void destroyMethod() {System.out.println("MyBean: 自定义的销毁方法");}// 获取消息的方法public String getMessage() {return message;}
}
配置类
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;@Configuration
public class AppConfig {@Bean(initMethod = "initMethod", destroyMethod = "destroyMethod")public MyBean myBean() {MyBean bean = new MyBean();bean.setMessage("Hello, Spring!");return bean;}
}
测试类
import org.springframework.context.annotation.AnnotationConfigApplicationContext;public class Main {public static void main(String[] args) {// 创建 Spring 容器AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);// 获取 BeanMyBean myBean = context.getBean(MyBean.class);System.out.println("MyBean: 使用 - message = " + myBean.getMessage());// 关闭容器,触发 Bean 的销毁过程context.close();}
}
代码解释
2.1 MyBean
类
- 构造函数:在 Bean 实例化时调用。
setMessage
方法:用于为 Bean 的属性赋值。@PostConstruct
注解的方法:在属性赋值后、初始化方法之前调用。afterPropertiesSet
方法:实现InitializingBean
接口的方法,在@PostConstruct
方法之后调用。initMethod
方法:自定义的初始化方法,在afterPropertiesSet
方法之后调用。@PreDestroy
注解的方法:在容器关闭前、销毁方法之前调用。destroy
方法:实现DisposableBean
接口的方法,在@PreDestroy
方法之后调用。destroyMethod
方法:自定义的销毁方法,在destroy
方法之后调用。
2.2 AppConfig
类
@Bean
注解:用于定义一个 Bean,initMethod
和destroyMethod
属性分别指定了自定义的初始化方法和销毁方法。
2.3 Main
类
- 创建
AnnotationConfigApplicationContext
容器,并加载AppConfig
配置类。 - 获取
MyBean
实例并使用。 - 关闭容器,触发 Bean 的销毁过程。
输出结果为:
MyBean: 实例化
MyBean: 属性赋值 - message = Hello, Spring!
MyBean: @PostConstruct 注解的初始化方法
MyBean: InitializingBean 接口的 afterPropertiesSet 方法
MyBean: 自定义的初始化方法
MyBean: 使用 - message = Hello, Spring!
MyBean: @PreDestroy 注解的销毁方法
MyBean: DisposableBean 接口的 destroy 方法
MyBean: 自定义的销毁方法
总结:
Spring Bean 的生命周期是一个复杂而有序的过程,通过实现不同的接口和使用注解,我们可以在 Bean 的不同阶段插入自定义的逻辑。理解 Bean 的生命周期有助于我们更好地控制 Bean 的创建、初始化和销毁过程,提高应用程序的性能和可维护性。